Type members
Types are comprised of properties, events and methods, collectively known as type members.
The following sections describe each different member type using examples from the builtin types. In addition to describing the different members, they show you how to read the Zing reference documentation for each.
The way members are declared in the documentation might seem strange at first, but as you'll learn later in the Guide: User elements chapter, it matches the way you will declare new members in code when building your own elements.
Properties
Properties store a value.
Properties can either be writable or read-only. Writable properties can be read from and written to. Read-only properties can be read from, but not written to.
Writable properties
Most properties can be both read and written. An example is the Material.Slider.value property shown below.

The documentation of writable properties usually also includes their default value. In the case of the value property, the default value is 0. If the default value is not a simple constant, it will be described in the documentation body.
Writable properties can be assigned a value or expression using the standard element declaration syntax, or assigned a value explicitly from code.
Material.Slider as slider { value: 0.5 } Material.Button { on activated { slider.value = 0.5 } }
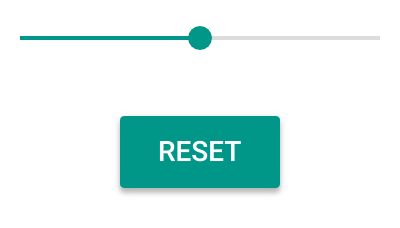
Read only properties
Read only properties cannot be written to. They are used by types to expose a value that cannot be changed directly.
For example, the PagedView.pageCount property is read only. Read only properties have a "read only" badge in the documentation.

While you cannot assign a value or expression to a read only property, they can still be used in other property expressions. For example, the following code displays the number of pages in a text label.
Text { text: "Pages: {0}".arg(pagedView.pageCount) }
Alias properties
New elements are often built by combining existing elements together. For example, the Apple.TabView element is a composite element, built out of TabView and Apple.TabBar elements.
A composite element is usually a "black box". There is no way to reach inside it and access the individual pieces. However, when required, composite elements can expose their inner constituent elements using alias properties. Exposing an inner element allows access to its properties, methods and events.
For example, the Apple.TabView.tabBar alias property looks like this.

The following code shows how the Apple.TabBar's style and showSeparator properties can now be assigned an expression.
Apple.TabView { tabBar.style: .Black tabBar.showSeparator: false }
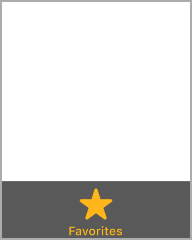
New children can also be added to an inner elements exposed using aliasing. This is so useful that some elements alias inner elements as a way to provide a "block" used to automatically position additional items.
Consider the Apple.NavigationBar.right property.

To add the accessory, open the alias block as though it was an element, and declare the child.
Apple.NavigationBar { right { Apple.Button { label: "Done" } } }
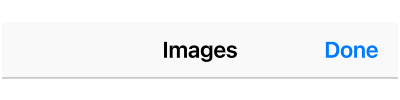
Events
Events notify you when something happens, such as a button being pressed or a timer elapsing. Event members are only available on element types.
A simple example is the Material.Button.activated() event, the documentation for which is shown below.

To respond to an element event, you write an event handler. An event handler is a block of code that is run when the event is emitted.
Material.Button { on activated { System.log("Button activated!") } }
Events can also have parameters, which pass extra information that you can access in an event handler.
For example, the the Gesture.tapped() event includes a single argument.

To access the parameter value, you add a receiver argument name to the event handler. The receiver name is up to you and doesn't have to match the name used in the documentation.
Rectangle { on Gesture.tapped(point) { System.log("Rectangle tapped at", point) } }
Methods
Methods are called to make an element or value type do something, or to calculate a value.
Methods take zero or more mandatory parameters and, optionally, return a value. The parameters and return value for each method are shown in the method documentation.
For example, the documentation for the Video.seekTo() method is reproduced below. The seekTo method takes a single argument, and does not return a value.

Calling the seekTo method looks like this.
video.seekTo(45)
A method's return value appears at the end of the method signature. As an example, the DateTime.toLocal() method documentation is shown below.

The toLocal method does not take any parameters, so calling it looks like this.
var localDate = date.toLocal()
Overloads
Some methods have multiple overloads. An overloaded method is one where there are multiple choices for its parameters. Zing selects the correct method to call based on the number and type of the parameters you pass.
For example, the String.mid() method has two overloads, the documentation for which is shown below.
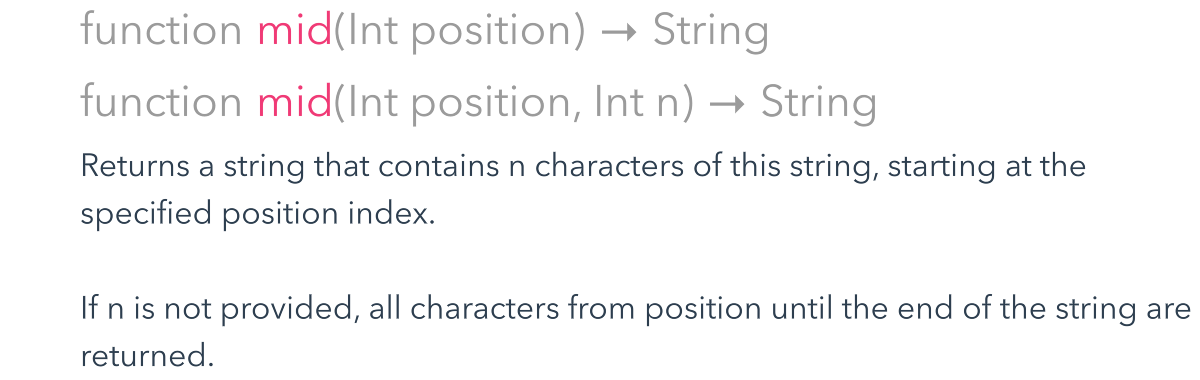
Calling each of the mid overloads looks like this.
var string = "The dog ate my homework!" var result1 = string.mid(15) // "homework!" var result2 = string.mid(4, 3) // "dog"
Optional parameters
Optional method parameters are used to pass extra information to the method. When the parameter is not provided, a default value is used instead.
Optional parameters are also called "named" parameters, because the parameter name is used to distinguish one from another. Optional parameters must be passed after any mandatory parameters, but if you pass more than one, their relative order doesn't matter.
The method's documentation will list the available optional parameters, along with their default values. For example, the Image.crossfade() method documentation is reproduced here.
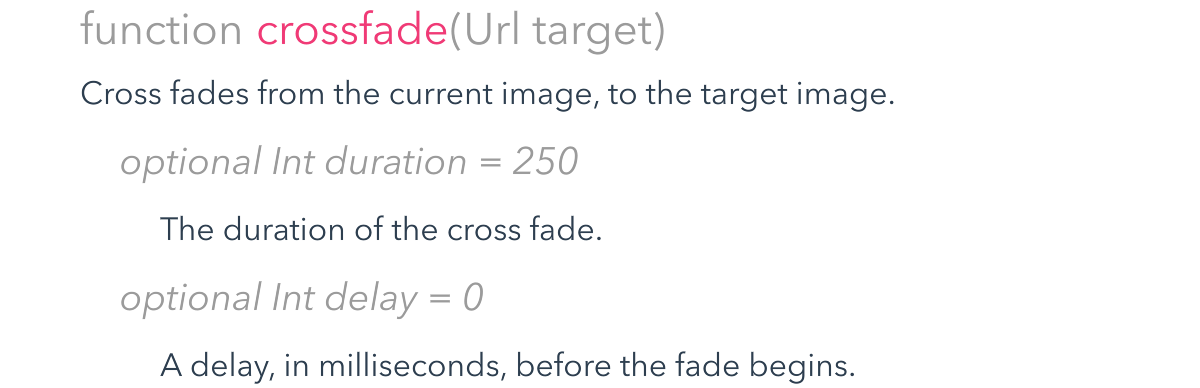
Three example crossfade calls are shown below.
image.crossfade("paris.jpg") image.crossfade("paris.jpg", duration: 500) image.crossfade("paris.jpg", delay: 100, duration: 500)
Static members
Static members are a type of global member that is associated with an element or value type.
When a member of a type is static it means that no matter how many instances of the type are created, there is only one copy of the static member. A static member is shared by all instances of the type.
The static Color.rgb() method is shown below.

As they aren't bound to a specific instance, static members are accessed by prefixing their associated type name.
var color = Color.rgb(0.33, 0.7, 0.33)
The builtin types mostly limit their use of static to methods and constants. However, static properties and events can also be useful for global communication and data in your prototypes. This is covered further in Guide: Static properties.
Extension members
Extension members are a type of global member that is settable on any element. In essence, extension members allow one element to "extend" another element by adding members to it.
One use of this is to allow child elements to specify individual values for properties that provide a parent with additional information on how they are to be presented. For example, the Apple.TabView element declares a tab extension property that allows each page to control the appearance of its tab bar item.

As with static properties, the extension type name is used as a prefix to qualify the property name. However, as they are still an instance property, extension properties are set on element instances.
Apple.TabView { Rectangle { Apple.TabView.tab.label: "News" } Rectangle { Apple.TabView.tab.label: "History" } }
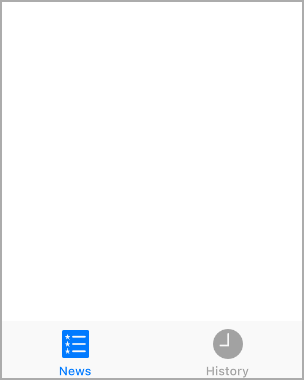
Context matters
One potentially confusing aspect of extension properties is their contextual influence.
An extension property can be set on any element regardless of its type or how it is being used, but it generally only has an effect in some contexts and on some types. If it is used outside of one of those contexts, it will simply have no effect.
For example, you can use the Apple.TabView.tab extension property on any element, but it will only have an effect if set on an Item that is used as an Apple.TabView page.