Extends Item
Text
The text element is for static text.
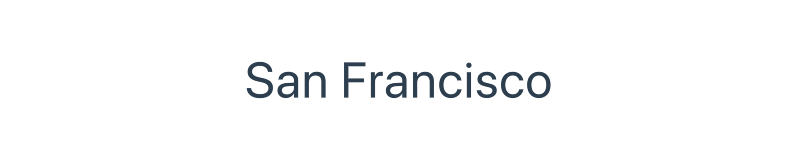
Text { text: "Hello world" font: "Helvetica Neue Light" size: 30 }
property Text.Alignment alignment: .Left
Sets the text's horizontal alignment.
Text.Alignment.Left
Text is aligned against the left edge.
Text.Alignment.Center
Text is centered in the item.
Text.Alignment.Right
Text is aligned against the right edge.
Text.Alignment.Justify
Text is justified.
The following example shows the effects of each alignment. Tap on the text to switch between the four options.
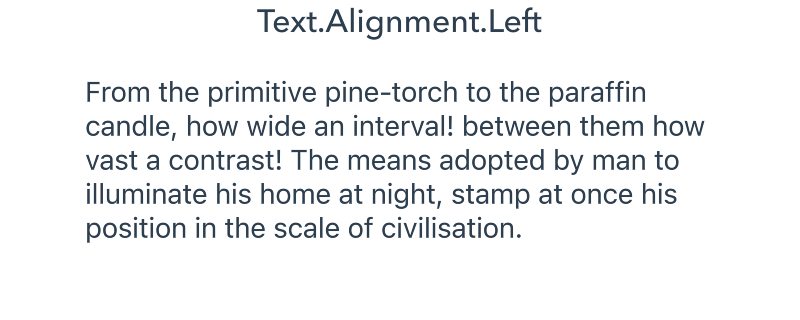
A color used to set the color of the displayed text.

Text { font: "Futura Medium" color: #99DE93 text: "It's full of stars!" }
property String font: "San Francisco"
A string used to specify the exact font. Fonts are not specified by family name with a separate weight. Instead the exact font, is specified e.g. "Helvetica Neue Light" or "Roboto Bold"
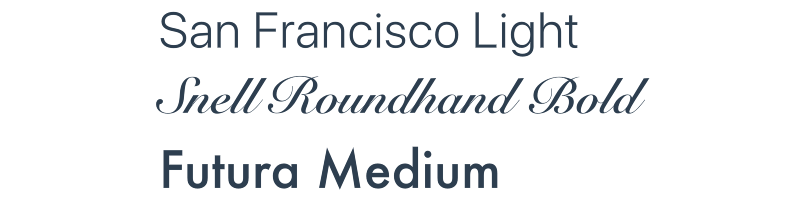
Text { text: font font: "Futura Medium" size: 20 }
property Float layoutWidthread only
property Float layoutHeightread only
The layoutWidth is the maximum width of the visible text. The layoutHeight is the maximum height of the visible text. If the width/height properties are not set they automatically resize to be the same as the layoutWidth and layoutHeight.
property Float maximumWidth: Int.Max
The maximum width of the displayed text. The text will grow to this width and then either wrap, or truncate depending on the mode.
property Float lineSpacing: 1.0
This property is used to define a regular spacing between each line.
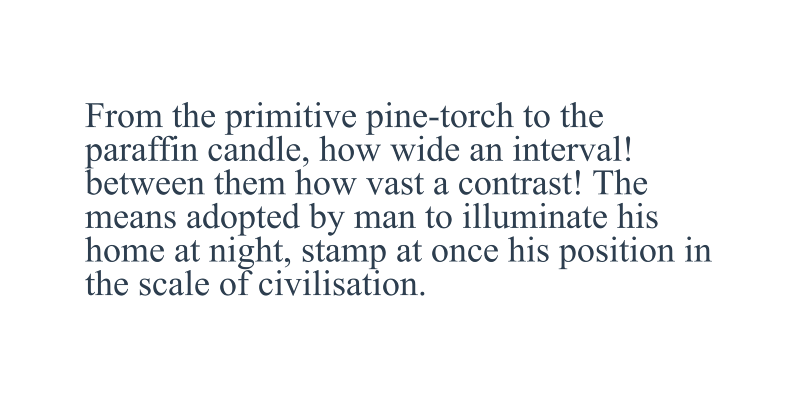
Text { width: 100 text: "From the primitive pine-torch to the paraffin candle, how" lineSpacing: 1.2 }
property Text.WrapMode wrapMode: .Word
Controls how the text wraps when it exceeds the width of the Text item.
Text.WrapMode.None
Do not wrap.
Text.WrapMode.Word
Wrap on word boundaries, or on grapheme bounds if needed.
Text.WrapMode.SingleLine
Treat the text as a single line with no wrapping, even if it exceeds the item's width or contains explicit carriage returns.
property Text.TruncateMode truncateMode: .None
Controls how the text is truncated if it exceeds the space available in the Text item.
Text.TruncateMode.None
The text is not truncated, but it is still clipped to the bounds of the Text item. The clipping may obscure part of a character or line.
Text.TruncateMode.Ellipsis
The text is truncated to fit the bounds of the Text item, and a trailing ellipsis is added if the truncation occurs mid paragraph.
property Bool preciselyBoundContent: false
Controls whether the Text item's implicit size is precisely bound to the text's layout size, or whether it is rounded to an integer boundary.
Generally the precise layout bounds of text are not round numbers. By default, the Text element rounds the text bounds up to the nearest integer. If this property is set to true, the Text element will use the precise layout values instead, which will likely be fractional numbers.