Extends Item
Camera
The Camera element displays the camera viewfinder.
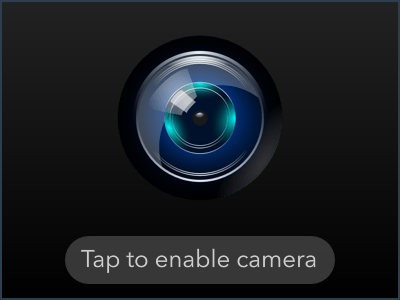
Camera { width: 200 height: 150 enabled: true }
When true, the Camera element is enabled and will load and display the viewfinder.
The camera consumes power, so should only be enabled when it is actually in use.
After the camera is first enabled, there is momentary delay before the viewfinder is displayed and the camera is ready to take pictures. The status property can be used to determine when the camera is ready.
Camera { width: 300 height: 150 enabled: true }
By default the Camera element will use the main rear camera. To use the front camera set the front property to true.
Camera { width: 300 height: 150 front: true enabled: true }
property Camera.Status statusread only
The status of the Camera.
Camera.Status.Idle
The element is disabled.
Camera.Status.Loading
The camera is enabled but still loading. It is not yet ready to display the viewfinder or take pictures.
Camera.Status.Ready
The camera is ready. It is displaying the viewfinder and is able to take pictures.
Camera.Status.Failed
The Camera has failed to start, either because there is no camera present or an error occurred.
property Camera.FillMode fillMode: .Cover
When the size of the Video item is different from the size of the video, the fillMode controls how the video fills the space available to it.
Camera.FillMode.Stretch
The camera image will be stretched to fit the exact width and height of the item, even if this distorts the image.
Camera.FillMode.Fit
Scales the camera image by its aspect ratio to fit the item. This may add padding around the image, but will not crop it.
Camera.FillMode.Cover
Scales the camera image by its aspect ratio to completely fill the item. This might result in some of the image being cropped out.
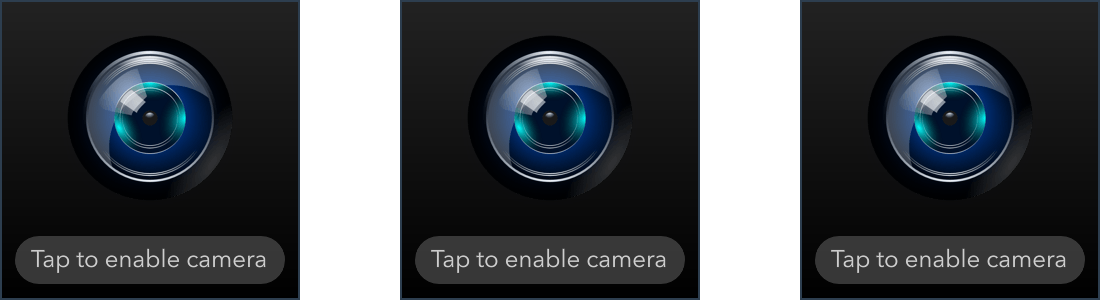
Camera { width: 400 height: 100 enabled: true fillMode: Camera.FillMode.Stretch }
function saveToGallery()
function saveToGallery(String gallery)
This method is used to capture a full sized image to the specified photo gallery, or the default camera roll if no gallery is specified.
In the desktop simulator, this method saves the image to the desktop.
Camera as camera { width: 300 height: 150 enabled: true with Gesture.Tap { on tapped { camera.saveToGallery("Zing") } } }