DateTime.Date
The DateTime.Date value type represents a calendar date.
A calendar, or "civil", date is the regular "year/month/day" method of representing a day in time, such as 2017/9/12. A civil date does not represent an unambiguous period of time as it varies depending on where you are in the world.
To represent an absolute time, a DateTime value should be used.
Operators
Subtracting one DateTime.Date from another returns the number of days between them.
var date1 = DateTime.Date(2019, 10, 21) var date2 = DateTime.Date(2019, 11, 6) Int days = date2 - date1 // 16
You can also add or subtract an integer number of days from a DateTime.Date.
var date = DateTime.Date(2017, 10, 9) DateTime.Date date2 = date + 9 // 2017-10-18
function DateTime.Date(Int year, Int month, Int date) → DateTime.Date
Return a new DateTime.Date for the specified calendar date.
static function DateTime.Date.today() → DateTime.Date
static function DateTime.Date.today(String timeZone) → DateTime.Date
static function DateTime.Date.today(DateTime.TimeZone timeZone) → DateTime.Date
Returns the current date for the specified time zone. If time zone is not provided, the local time zone is used.
See DateTime.TimeZone for a description of the time zone format.
property Bool isValidread only
True if this is a valid date, false otherwise. A DateTime.Date is invalid if it has not been assigned a value. Modifying an invalid DateTime.Date in any way will make it valid.
property Int dayOfWeekread only
property Int dayOfYearread only
The calendar year, month, day, day of the week and day of the year of the DateTime.Date.
The year is the complete year value, such as 2016. The month ranges from 1-12, and the date from 1-31.
The dayOfWeek ranges from 0-6, with 0 corresponding to Sunday and 6 to Saturday. The dayOfYear ranges from 1-365 (or 1-366 on leap years).
function time(Int hour, Int minute, Float second) → DateTime
function time(Int hour, Int minute, Float second, String timeZone) → DateTime
function time(Int hour, Int minute, Float second, DateTime.TimeZone timeZone) → DateTime
Returns a DateTime value for the specified time on the current date. If a time zone is not provided, the local time zone is used.
See DateTime.TimeZone for a description of the time zone format.
function format(String pattern) → String
Returns the formatted date string using the provided pattern.
The pattern string may contain any of the tokens shown below. Each matching token in the pattern is replaced with the corresponding date component value.
var date = DateTime.Date(2017, 10, 9) // 2017-10-09 System.log(date.format("YYYY-MM-DD"))
Characters in the pattern that do not form part of a token are left as is. However, as new tokens may be added in the future, it is safest to enclose these "pass through" characters in single quotes to ensure they are never treated as a pattern token.
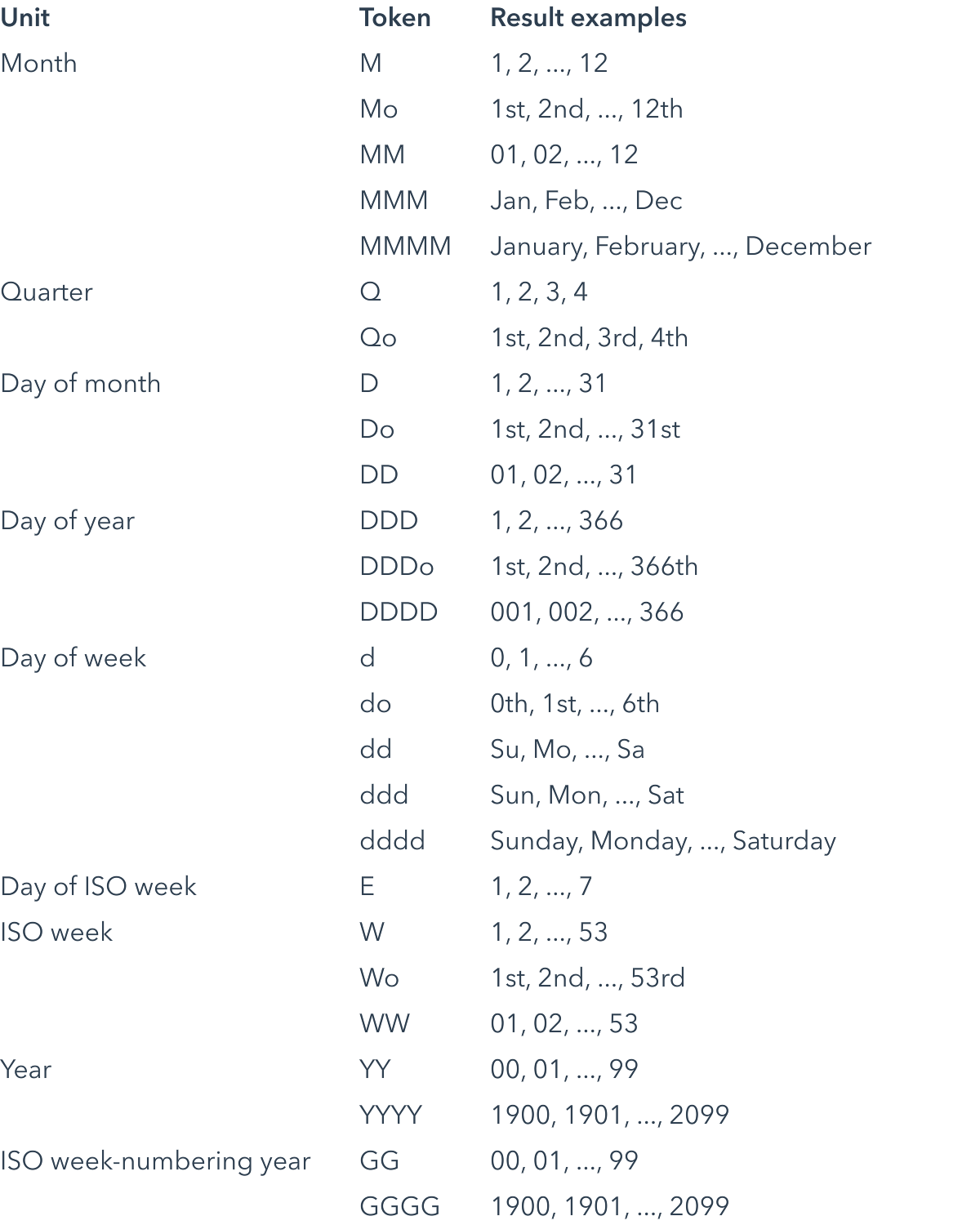