Extends Animation
Animation.Tween
Tween animations interpolate a property between two values. Animation.Tween supports tweening properties of type Float, Int or Color.
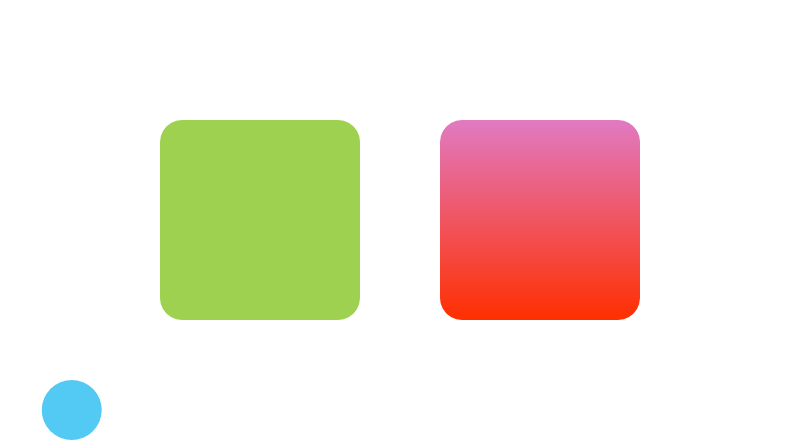
Rectangle as rect { width: 100 height: 100 color: #orange } Animation.Tween { running: true target: rect.color to: #green duration: 3000 }
property ref target
A reference to the property to be animated. The type of the targetted property, either Float, Int or Color, dictates the valid values for the from and to properties.
For example, the following example targets a Color property, so the to and from values are colors.
Animation.Tween { target: rect.color from: #blue to: #green duration: 3000 }
Whereas the next example targets a Float property, so the to and from values are numbers.
Animation.Tween { target: rect.width from: 100 to: 150 duration: 3000 }
property var from
property var to
The from and to values to interpolate between. If from is not set, then the value of the target property when the animation begins will be used.
Animation.Tween { target: rect.color from: #blue to: #green duration: 3000 }