Extends Item
PagedView
PagedView provides a horizontal, paged model view of multiple pages that allows the user to swipe left and right between them. Each page in the PagedView is automatically sized to fill the view itself.
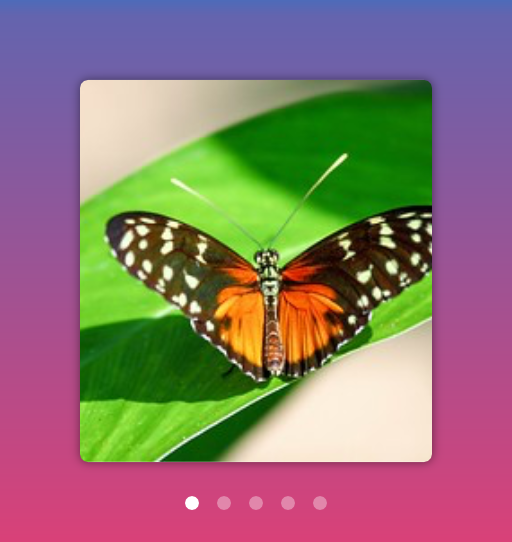
PagedView { Rectangle { color: #red } Rectangle { color: #green } Rectangle { color: #blue } }
Adding and removing pages
As shown above, each inline child item automatically becomes a PagedView page.
To add and remove additional pages using code, call the PagedView.addPage() and PagedView.removePage() methods.
Scrolling
The PagedView is only responsible for the horizontal paging. If you also need the individual pages to scroll, they need to either be wrapped in or contain a ScrollView.
PagedView { width: 320 height: 480 ScrollView { InfoPane { width: 320 } } ScrollView { InfoPane { width: 320 } } }
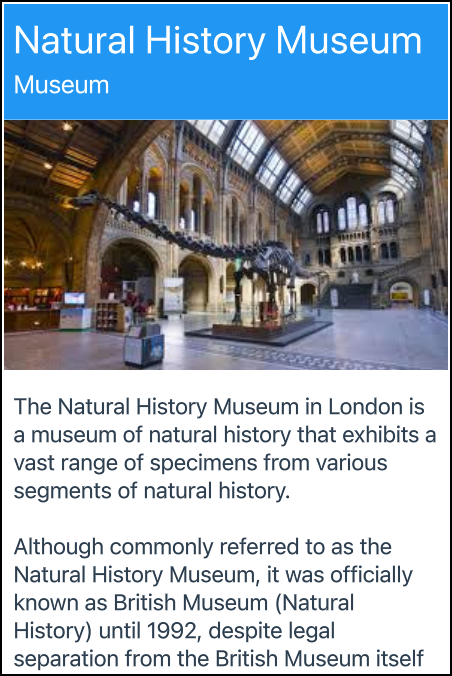
property Bool scrollable: true
If true scrolling is enabled, and if false scrolling is disabled.
If scrolling is disabled the PagedView ignores touch interaction. You can still programatically change pages using the jumpToPage() and scrollToPage() methods.
property PagedView.Orientation orientation: .Horizontal
The scroll orientation of the PagedView pages.
PagedView.Orientation.Horizontal
Horizontal pages that scroll left to right.
PagedView.Orientation.Vertical
Vertical pages that scroll top to bottom.
property PagedView.PageIndicatorStyle pageIndicatorStyle: .None
Sets the appearance of the page indicator. You can also build custom indicators using the page and pageCount properties.
PagedView.PageIndicatorStyle.None
No page indicators are displayed.
PagedView.PageIndicatorStyle.Black
Black page indicators are displayed.
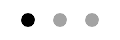
PagedView.PageIndicatorStyle.White
White page indicators are displayed.
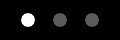
property Float pageIndicatorInset: 8
The inset, from the bottom of the view's safe area, at which the page indicator is shown.
function removePage(Item page)
Add and remove pages from the PagedView.
You can only remove a page that was previously added by calling addPage().
function jumpToPage(Int pageIndex)
Jump immediately to the specified page. If you would like to animate to a page, use scrollToPage() instead.
function scrollToPage(Int pageIndex)
Animate to the specified page. If you would like to jump immediately to a page, use jumpToPage() instead.