Item
The Item element is the base of all elements that appear on the Zing canvas.
Item doesn't paint any content itself, but many of the elements that derive from Item do. The common Item properties define the geometry, transform, stacking and clipping which control how painted content appears on the canvas.
Define the geometry of the Item.
The x and y values are relative to the transform of the items visual parent.
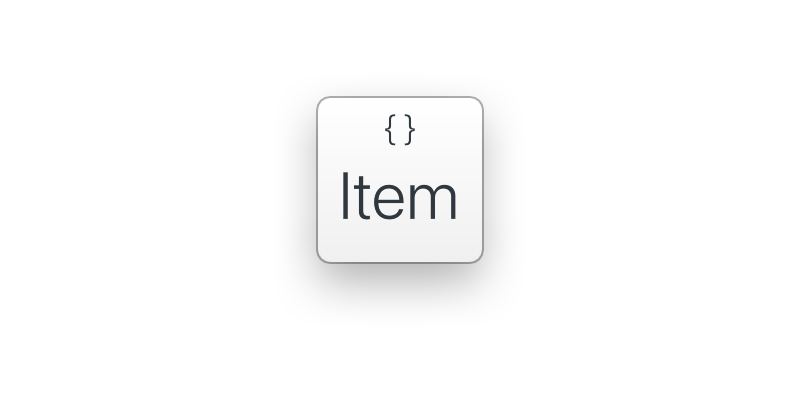
When set, the visual contents of the item and its children are clipped to the item's shape. By default, the shape of an item is the rectangle defined by its width and height.
Special clipping is applied to Rectangles with rounded corners and Ellipses, where the content is clipped to the curved edges instead.
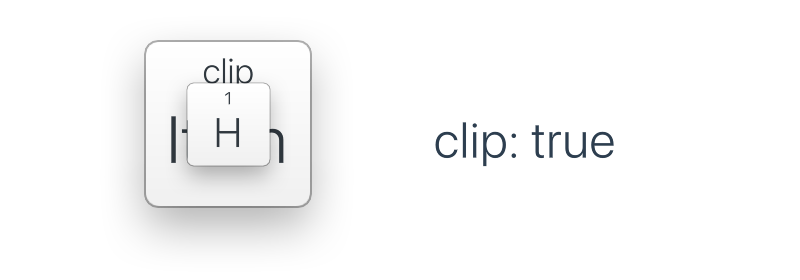
The Item's opacity, ranging from 0.0 (transparent) to 1.0 (opaque). A value of 0.5 corresponds to 50% opacity.
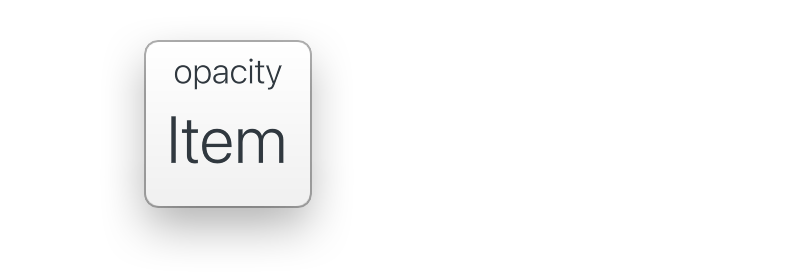
Define the rotation and scale of the Item.
The scale and rotation are applied around the item's transformOrigin, which is one of 9 predefined grid points. By default, the transform origin is the item's center.
Rotation is specified in degrees clockwise.
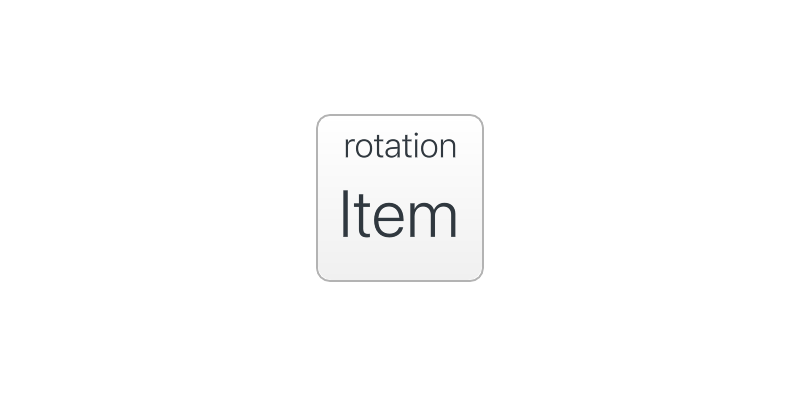
property Item.TransformOrigin transformOrigin: .Center
When items are rotated or scaled they do so about the transform origin. The transform origin can be one of the following 9 points.
Item.TransformOrigin.Center
Item.TransformOrigin.TopLeft
Item.TransformOrigin.Top
Item.TransformOrigin.TopRight
Item.TransformOrigin.Left
Item.TransformOrigin.Right
Item.TransformOrigin.BottomLeft
Item.TransformOrigin.BottomRight
Item.TransformOrigin.Bottom
Click the origin point below to see the effect it has on the transformation.
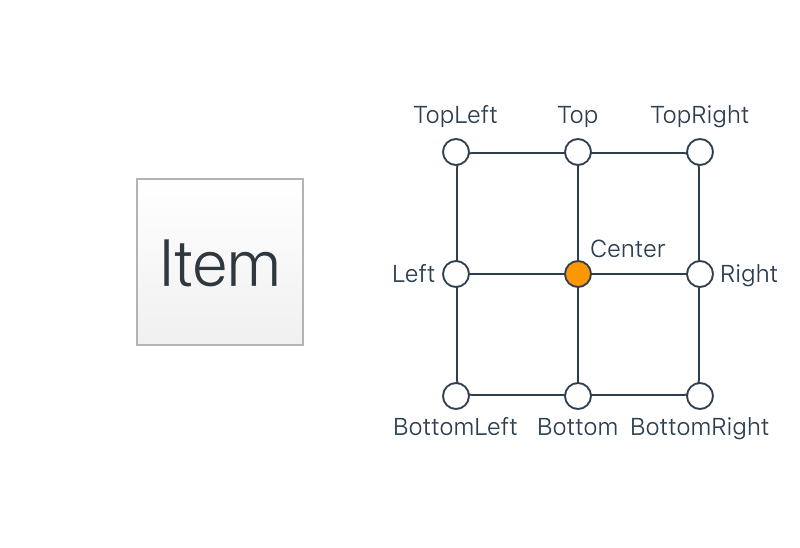
The following example sets the tranform origin point to the top left.
Item { transformOrigin: .TopLeft }
The item's stacking value.
The stacking value, along with the item's child order, controls the order in which the item is drawn relative to its siblings. An item with a higher stacking value will be "above" its siblings with lower stacking values.
For a complete explanation of stacking in Zing, see Guide: Children.
function takeFocus()
function clearFocus()
function clearFocus(Item.ClearFocusMode mode)
Keyboard focus is an important feature of any application as it controls where keyboard input is directed, and when the keyboard itself is shown.
Item.ClearFocusMode.SingleItem
Item.ClearFocusMode.IncludeChildren
delegate preferredFocusDelegate() → Item
event focusChangedEvent(Item.FocusEvent e)
function mapToItem(Item toItem, Vec2 point) → Vec2
function mapToItem(Item toItem, Float x, Float y) → Vec2
function mapFromItem(Item fromItem, Vec2 point) → Vec2
function mapFromItem(Item fromItem, Float x, Float y) → Vec2
These methods transform a point from one item's coordinate system another.
mapToItem() takes a point in this items coordinate space, and returns the equivalent point in the toItem's coordinates.
mapFromItem() takes a point in fromItem's coordinate space, and returns the equivalent point in this item's coordinates.
function mapToGlobal(Vec2 point) → Vec2
function mapToGlobal(Float x, Float y) → Vec2
function mapFromGlobal(Vec2 point) → Vec2
function mapFromGlobal(Float x, Float y) → Vec2
These methods transform a point to and from the "global" coordinate space. If the item is part of the visual hierarchy, the "global" coordinate space is the screen. If not, it is the outer coordinate space of the item's greatest ancestor.
property Array<Item> childrenread only
The visual children of the Item.
You cannot modify the children array directly. To add or remove children, use the Item.setContainer() method.
To modify the order of the children list, use the item ordering functions: Item.orderFirst(), Item.orderLast(), Item.orderAfter() and Item.orderBefore().
function setContainer(Item targetItem)
function changeContainer(Item targetItem)
Changes the item's container to the specified target.
changeContainer() will also attempt to update the item's Item.x, Item.y, Item.scale and Item.rotation properties to ensure that the item remains in the same global screen position. That is, such that it doesn't appear to visually "move".
function clearChildren()
Remove all the visual children of this item. This is equivalent to setting the container for each child to null.
function orderFirst()
function orderLast()
function orderBefore(Item beforeItem)
function orderAfter(Item afterItem)
These methods modify the position of the item in its container's Item.children list. That is, they modify the item's "child order".
orderFirst() and orderLast() move the item to the beginning or end in its current container.
orderBefore() and orderAfter() move the item to immediately before or after the specified item.
The child order, along with the item's Item.stacking value, controls the order in which the item is drawn relative to its siblings. An item with a higher child order will be "above" its siblings with a lower child order.
For a complete explanation of stacking in Zing, see Guide: Children.
property Float safeAreaInsetLeftread only
property Float safeAreaInsetRightread only
property Float safeAreaInsetTopread only
property Float safeAreaInsetBottomread only
Warning
It is extremely easy to misuse these properties. Whenever possible, use anchors for layout and don't rely on the safe area inset values.
The Item's safe area, expressed as insets from its boundary.
function isAncestorOfMe(Item other) → Bool
function isDescendentOfMe(Item other) → Bool
Returns true if other is an ancestor or descendent of this item.
optional Bool proper = false
When true, other must be a "proper" ancestor or descendent (that is, not this item). If false, an item is considered both an ancestor and a descendent of itself.
static function Item.commonAncestor(Item item1, Item item2) → Item
Returns the first common ancestor of both item1 and item2, or null if there is no common ancestor.
optional Bool proper = false
When true, the returned node will be the first "proper" ancestor node rather than either item1 or item2 themselves.
static function Item.focusItem() → Item
Returns the currently focused item, or null if no item has focus.
static event Item.unhandledKeyEvent(Keys.Event event) → Bool
The unhandledKeyEvent event provides a global way to monitor all unhandled key events. It is emitted when a key event, such as a press or release, is not otherwise handled.
To accept the key event, return true from the handler. To ignore it return false.