Regex
Regex is a value type for matching regular expressions.
Zing Studio uses the Google RE2 regular expression engine. The particular regular expression syntax accepted by this engine is documented on their wiki page.
You can try out regular expressions and see the results below.
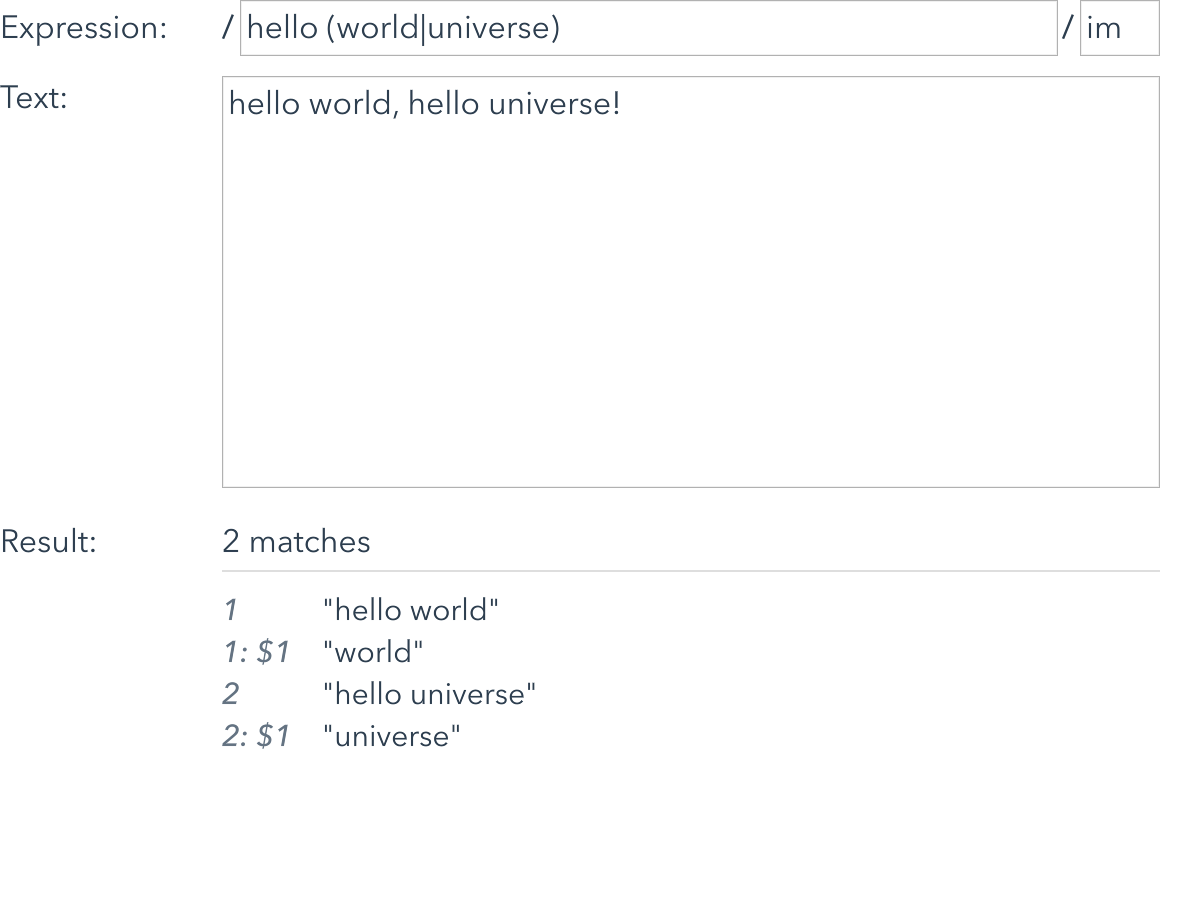
Literal syntax
Regular expression literals can be written directly, enclosing the pattern within two '/' characters.
var regex = /hello (world|universe)/
Where possible, you should always prefer Regex literals over explicitly creating Regex objects as literals are easier to write, give better error reporting and are faster to create.
Modifier flags
You can include optional flags following the regular expression to modify its behavior. Currently the following flags are supported.

For example, to create a case insensitive regex you add the "i" modifier flag to the declaration.
var regex = /hello (world|universe)/i
function Regex(String pattern) → Regex
function Regex(String pattern, String flags) → Regex
Return a new Regex for the specified pattern and flags.
var regex = Regex("hello (world|universe)")
If you pass an invalid pattern or an invalid flag, the Regex.errorDescription property will be set.
Where possible, use Regex literals instead of explicitly creating Regex objects as literals are easier to write, give better error reporting and are faster to create.
function test(String string) → Bool
Returns true if the regular expression matches the string.
if /dog|cat/.test("Who let the dogs out?") { // ... matched ... }
The test() method is often faster than match(), so should be used if the additional information provided by match() isn't required.
function match(String string) → Regex.Match
Returns the first regular expression match in the string, or an invalid match value if there were no matches.
You can test Regex.Match value type's validity directly in flow control statements like this:
if var m = /dog|cat/.match("Who let the dogs out?") { System.log("Someone let the", m.string, "out") }
function matches(String string) → Array<Regex.Match>
Returns all the regular expression matches in the string.
You can use matches() to efficiently iterate over all the matches in a given string.
The following example sums all the integer values in a text document.
var total = 0 for var m in /^value: (\d+)$/m.matches(text) { total += m.groups[1].string.toInt() } System.log("Total is", total)