Extends Item
ScrollView
The ScrollView element allows an application to display content that is larger than the size of the application's window by letting users to scroll around using swiping gestures.
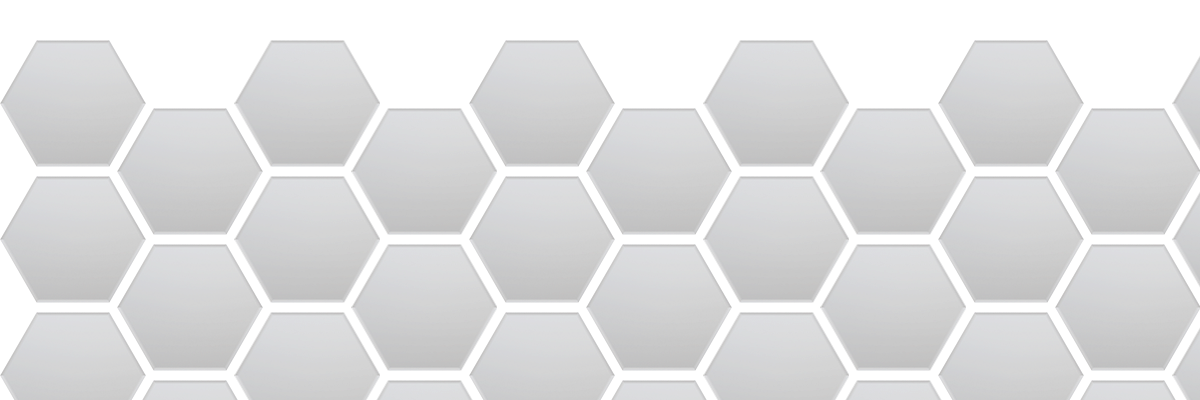
property Bool scrollable: true
If true scrolling is enabled, and if false scrolling is disabled.
If scrolling is disabled the ScrollView ignores all touch events.
property ScrollView.Orientation orientation: .HorizontalAndVertical
Sets the allowable scroll orientations.
ScrollView.Orientation.Horizontal
Allow horizontal scrolling.
ScrollView.Orientation.Vertical
Allow vertical scrolling.
ScrollView.Orientation.HorizontalAndVertical
Allow both horizontal and vertical scrolling.
If true the scroll area bounces when it encounters a boundary of the content. Bouncing visually indicates that scrolling has reached the edge of the content. If false, scrolling stops immediately at the content boundary.
property Bool alwaysBounceX: false
property Bool alwaysBounceY: false
By default, ScrollView will only allow dragging when the content region is larger than the bounds of the ScrollView. For example, if the ScrollView's contentWidth is less than its width, horizontal dragging will be disabled.
If these properties are set to true, and the bounces property is also true, dragging will be allowed even when the content is smaller than the bounds of the ScrollView.
property Float contentWidth: 0
property Float contentHeight: 0
Explicitly defines the bounding rectangle of the content to scroll.
By default the ScrollView uses the bounding rectangle of its children, so setting these properties is usually not required. However, to override the default and provide a custom content rectangle, you can set the contentX, contentY, contentWidth and contentHeight properties manually.
Setting any one of these properties explicitly disables the automatic child rectangle logic. For example, if you set contentWidth explicitly, the contentHeight would be treated as 0 unless you also explicitly set it.
property Float indicatorInsetLeft
property Float indicatorInsetRight
property ScrollView.ContentInsetAdjustmentStyle contentInsetAdjustmentStyle: .OrientationAxes
ScrollView.ContentInsetAdjustmentStyle.Never
ScrollView.ContentInsetAdjustmentStyle.Always
ScrollView.ContentInsetAdjustmentStyle.OrientationAxes
property ScrollView.IndicatorStyle indicatorStyle: .Default
Sets the appearance of the scroll indicators.
ScrollView.IndicatorStyle.None
Do not show scroll indicators.
ScrollView.IndicatorStyle.Default
A light gray scroll indicator.
ScrollView.IndicatorStyle.Black
A darker gray scroll indicator.
ScrollView.IndicatorStyle.White
A white scroll indicator.
property Color androidOverScrollTint: #gray700
The tint color used in the Android over scroll edge effect.
Enables or disables paging. When enabled, the ScrollView stops on multiples of the ScrollView's bounds when the user scrolls.
property Bool scrollsToTop: false
Controls whether the scroll-to-top gesture is enabled for this ScrollView.
The scroll-to-top gesture is a tap on the status bar on iOS devices. When a user makes this gesture, the system asks the ScrollView closest to the status bar with the scrollsToTop property set to scroll to the top.
On iPhone, the scroll-to-top gesture has no effect if there is more than one ScrollView on screen that has scrollsToTop set.
property Bool trackingread only
property Bool draggingread only
property Bool deceleratingread only
Together the tracking, dragging and decelerating properties allow you to track the interaction state of the ScrollView.
The tracking property is true whenever the user is touching the ScrollView. Tracking returns to false once the user releases the ScrollView, or the interaction is canceled for some other reason.
The dragging property is true when the ScrollView is being dragged by the user. Whenever dragging is true, tracking will also be true. Dragging returns to false once the user releases the ScrollView, or the interaction is canceled for some other reason.
The decelerating property is true whenever the ScrollView is animating. This may be because the user flicked the ScrollView, it is automatically scrolling back to a valid position, or the scrollToOffset() method has been called.
property Bool directionalLock: false
If false, scrolling is permitted in both horizontal and vertical directions. If true and the user begins dragging in one general direction (horizontally or vertically), the scroll area disables scrolling in the other direction. If the drag direction is diagonal, then scrolling will not be locked and the user can drag in any direction until the drag completes.
property Float offsetXread only
property Float offsetYread only
These are read only properties that specify the current offset of the scroll area content.
function ensureVisible(Item item)
function ensureVisible(Float x, Float y)
function ensureVisible(Float x, Float y, Float width, Float height)
If the specified item, point or rectangle is not within the scroll view's visible area, immediately move the ScrollView so that it is. If the item, point or rectangle is already visible, the ScrollView is not moved.
optional ScrollView.EnsureVisiblePosition moveTo = .Edge
ScrollView.EnsureVisiblePosition.Edge
Minimize the amount the ScrollView is moved, such that the target region is at the edge of the visible area.
ScrollView.EnsureVisiblePosition.Center
Scroll the view such that the target region is as close to the center of the visible area as possible.
function jumpToOffset(Float offsetX, Float offsetY)
Jump immediately to the specified offset. If you would like to animate to an offset, use scrollToOffset() instead.
optional Bool clampToBounds = true
If clampToBounds is set, the offset is clamped to the ScrollView's valid range. Otherwise, the content offset can be set to a value outside the valid range and the ScrollView will then animate it back into range.
optional Id id
If provided, the id will be passed to the ScrollView.scrollEvent()s generated for this interaction.
function jumpToTop()
function jumpToBottom()
Jump immediately to the top or bottom of the content area.
function scrollToOffset(Float offsetX, Float offsetY)
Animate the content area offset to specified value. If you would like to jump to an offset without animation, use jumpToOffset() instead.
optional Bool clampToBounds = true
If clampToBounds is set, the offset is clamped to the ScrollView's valid range. Otherwise, the content offset can be set to a value outside the valid range and the ScrollView will then animate it back into range.
optional Id id
If provided, the id will be passed to the ScrollView.scrollEvent()s generated for this interaction.
function jumpToItem(Item item)
function scrollToItem(Item item)
optional ScrollView.Position position = .Start
ScrollView.Position.Start
ScrollView.Position.Center
ScrollView.Position.End
optional Id id
If provided, the id will be passed to the ScrollView.scrollEvent()s generated for this interaction.
event scrollEvent(ScrollView.ScrollEvent e)
The scrollEvent event is emitted when the ScrollView scrolls. A scroll event can be caused by user interaction, programatically through API calls or because the ScrollView's bounds changed and it must scroll back into range.