Extends Item
Video
The Video element is for playing video.
Video support varies between operating systems. To ensure your project works on all plaforms, try to only use common video formats like MP4.
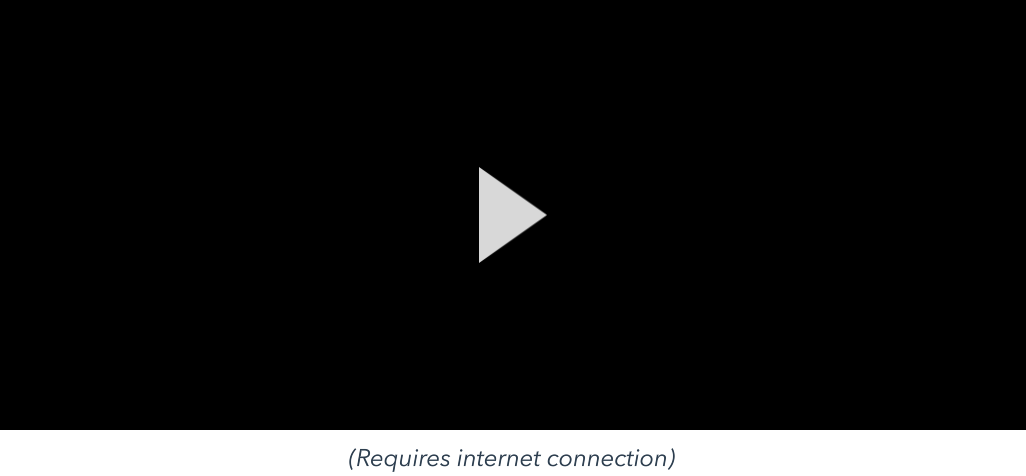
Video { source: "video.mp4" }
When true, the Video element is enabled and will preroll its video and respond to other commands, like play().
You might disable a video when you want to control exactly when it begins loading and consuming resources.
property Video.Status statusread only
The status of the Video.
Video.Status.Idle
The element is disabled, or there is no source specified.
Video.Status.Loading
The element is loading the video source.
Video.Status.Ready
The element has successfully loaded the video, and is ready for playback. The first frame of the video will be displayed.
Video.Status.Failed
The element has failed to load the video source.
The video's source url. This may be a local file in your project, or a network URL.
If you specify an audio file as the source, the audio will play without any video.
function play()
function pause()
function stop()
function seekTo(Float seconds)
Play, pause, stop and seek the video.
property Float durationread only
The current play time, and the total duration of the video in seconds.
You can change the play time by calling the seekTo() method.
property Int videoWidthread only
property Int videoHeightread only
The natural width and height of the video.
The volume at which to play the video, from 0.0 which mutes the video to 1.0 which plays it at maximum volume.
property Array<Video.TimeRange> bufferedTimeRangesread only
The time ranges for which content has been buffered.
property Video.TimeRange seekableTimeRangeread only
property Array<Video.TimeRange> seekableTimeRangesread only
The time ranges within the content that can be seeked to. In a local file, the seekable range will be the entire video, however in a live stream, the seekable range will often grow or change over time as new content is produced and older content is removed.
The seekableTimeRanges list contains the complete list of seekable time ranges. It is possible, although unusual, for this to be a discontiguous set of times. The seekableTimeRange property is a simplified time range that simply spans all the seekableTimeRanges including any gaps.
property Video.AudioCategory category: .Ambient
Controls how the video's audio affects background music apps the user has running, such as the Apple Music or Spotify apps.
Video.AudioCategory.Ambient
The audio is mixed with the background music.
Video.AudioCategory.AppMusic
Background music is paused when this Video plays.
In both cases, the Video is silenced if the user locks their device or exits the app.
property Video.FillMode fillMode: .Cover
When the size of the Video item is different from the size of the video, the fillMode controls how the video fills the space available to it.
Video.FillMode.Stretch
The video will be stretched to fit the exact width and height of the item, even if this distorts the picture.
Video.FillMode.Fit
Scales the video by its aspect ratio to fit the item. This may add padding around the video, but will not crop it.
Video.FillMode.Cover
Scales the video by its aspect ratio to completely fill the item. This might result in some of the image being cropped out.
property Video.ConfigurationHint configurationHints
The configuration hints property allows you to influence the configuration of the platform-specific media framework used to play the video.
These flags are just hints to the system. The specific behavior of each will vary between different platforms, and may have no effect at all on some devices.
Video.ConfigurationHint.PreferSoftwareCodec
Where possible, use a software-only codec for video playback.
While usually lower performance, this can be useful on resource limited platforms that place a constraint on the number of hardware accelerated videos that can be decoded simultaneously.