DateTime
The DateTime value type stores an absolute time and a time zone.
An absolute time is a concrete point in time, and is the same for everyone regardless of where they are in the world. For example, the moment man landed on the moon is an absolute time.
A civil time is the regular "year/month/day hours:min:seconds" method of representing a time, such as 2017/9/12 at 1:10pm. A civil time does not represent an unambiguous absolute time as it varies depending on where you are in the world.
A time zone describes how to convert an absolute time into a civil time and vice versa. For example, man landed on the moon 1969/07/20 at 4:18pm in the America/New_York time zone and 1969/07/21 at 6:18am in the Australia/Sydney time zone.
Internally a DateTime stores an absolute time and a specific time zone. When you access civil time properties, such as the hours or date properties, the time zone is used to convert the absolute time into a civil time. If you change the DateTime's time zone, using the toTimeZone() method, it will still refer to the same absolute time, but the civil time it returns will change.
Operators
Subtracting one DateTime from another returns the number of seconds between them.
var date1 = DateTime.time(7, 30, 0) var date2 = DateTime.time(8, 30, 0) Float seconds = date2 - date1 // 3600
You can use the subtraction operator to time how long something takes.
var startTime = DateTime.now() // Do some work System.log("Work took:", DateTime.now() - startTime)
Adding or subtracting a number from a DateTime adds or subtracts the specified number of seconds.
var date1 = DateTime.time(7, 30, 0) var date2 = date1 + 15 // 7:30:15
static function DateTime.now() → DateTime
static function DateTime.now(String timeZone) → DateTime
static function DateTime.now(DateTime.TimeZone timeZone) → DateTime
Returns the current date and time. If a time zone is not provided, the local time zone is used.
See DateTime.TimeZone for a description of the time zone format.
function time(Int hour, Int minute, Float second) → DateTime
static function DateTime.time(Int hour, Int minute, Float second) → DateTime
static function DateTime.time(Int hour, Int minute, Float second, String timeZone) → DateTime
static function DateTime.time(Int hour, Int minute, Float second, DateTime.TimeZone timeZone) → DateTime
Returns a DateTime for the specified time.
The instance variant uses the same date and time zone as the current DateTime.
The static variants all use the current date, and the time zone provided, or the local time zone if one is not provided.
See DateTime.TimeZone for a description of the time zone format.
function DateTime(DateTime.Date date) → DateTime
function DateTime(Int year, Int month, Int day) → DateTime
function DateTime(DateTime.Date date, String timeZone) → DateTime
function DateTime(DateTime.Date date, DateTime.TimeZone timeZone) → DateTime
function DateTime(Int year, Int month, Int day, String timeZone) → DateTime
function DateTime(Int year, Int month, Int day, DateTime.TimeZone timeZone) → DateTime
function DateTime(DateTime.Date date, Int hour, Int minute, Float second) → DateTime
function DateTime(DateTime.Date date, Int hour, Int minute, Float second, String timeZone) → DateTime
function DateTime(DateTime.Date date, Int hour, Int minute, Float second, DateTime.TimeZone timeZone) → DateTime
function DateTime(Int year, Int month, Int day, Int hour, Int minute, Float second) → DateTime
function DateTime(Int year, Int month, Int day, Int hour, Int minute, Float second, String timeZone) → DateTime
function DateTime(Int year, Int month, Int day, Int hour, Int minute, Float second, DateTime.TimeZone timeZone) → DateTime
Return a new DateTime for the specified civil date and time. If a time zone is not provided, the local time zone is used.
See DateTime.TimeZone for a description of the time zone format.
property Bool isValidread only
True if this is a valid date time, false otherwise. A DateTime is invalid if it has not been assigned a value. Modifying an invalid DateTime in any way will make it valid.
property Int dayOfWeekread only
property Int dayOfYearread only
The calendar year, month, day, day of the week and day of the year of the DateTime.
The year is the complete year value, such as 2016. The month ranges from 1-12, and the day from 1-31.
The dayOfWeek ranges from 0-6, with 0 corresponding to Sunday and 6 to Saturday. The dayOfYear ranges from 1-365 (or 1-366 on leap years).

The hour, minute, second and millisecond values of the DateTime.
Hour ranges from 0-23, minute from 0-59, second from 0-59 and millisecond 0-999.
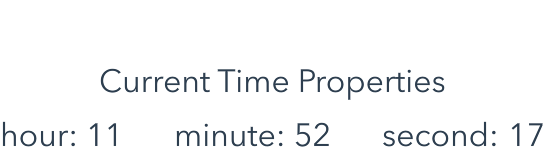
property DateTime.TimeZone timeZoneread only
The DateTime's time zone. To change time zones, use the toTimeZone() method.
function toTimeZone(String timeZone) → DateTime
function toTimeZone(DateTime.TimeZone timeZone) → DateTime
Returns an equivalent DateTime in the specified time zone. If an invalid time zone is provided, the UTC time zone is used.
See DateTime.TimeZone for a description of the time zone format.
function format(String pattern) → String
Returns the formatted date string using the provided pattern.
The pattern string may contain any of the tokens shown below. Each matching token in the pattern is replaced with the corresponding date component value.
var date = DateTime(2017, 10, 9, 15, 13, 32) // 2017-10-09 at 3.13.32 PM System.log(date.format("YYYY-MM-DD 'at' h.mm.ss A"))
Characters in the pattern that do not form part of a token are left as is. However, as new tokens may be added in the future, it is safest to enclose these "pass through" characters in single quotes to ensure they are never treated as a pattern token.
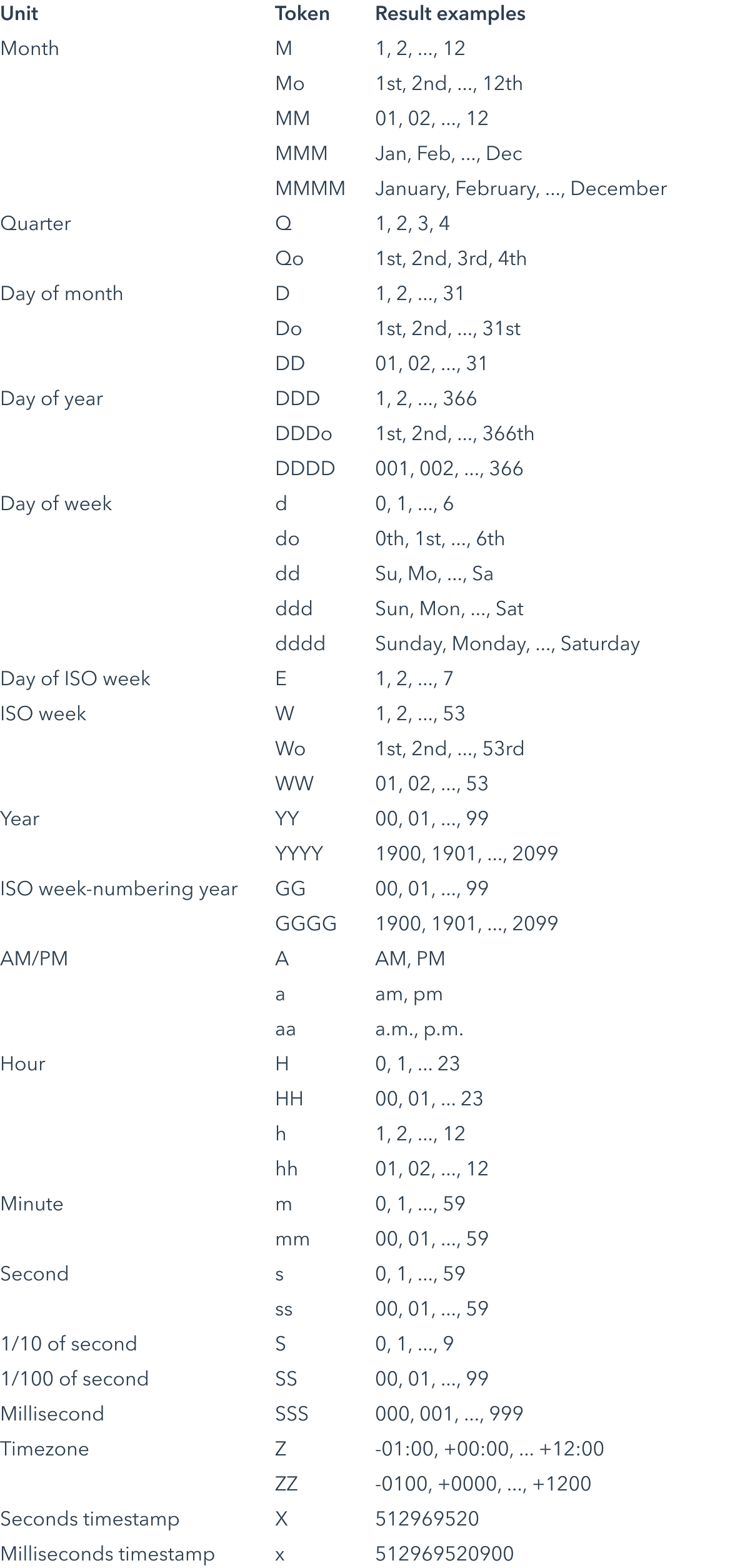
function toISOString() → String
static function DateTime.parseISOString(String string) → DateTime
Create or parse an ISO 8061 date string from a DateTime. If the parsed data does not contain an understood ISO string, an invalid DateTime is returned.
var date = DateTime.parseISOString("1879-03-14T11:30:00+01:00") System.log(date.toISOString())
static function DateTime.fromUnixTime(Float seconds) → DateTime
Convert to and from Unix time.
Unix time, also known as POSIX time or UNIX Epoch time, is the number of seconds that have elapsed since 00:00:00 Thursday, 1 January 1970, UTC, minus leap seconds.
DateTime values created from Unix time are in the UTC time zone by default. Use DateTime.toLocal() or DateTime.toTimeZone() to convert them to your local or target time zone.