Color
Color is a value type that stores RGBA colors.
Literal syntax
Colors are written starting with a # character followed by the color name. Zing defines the following colors:
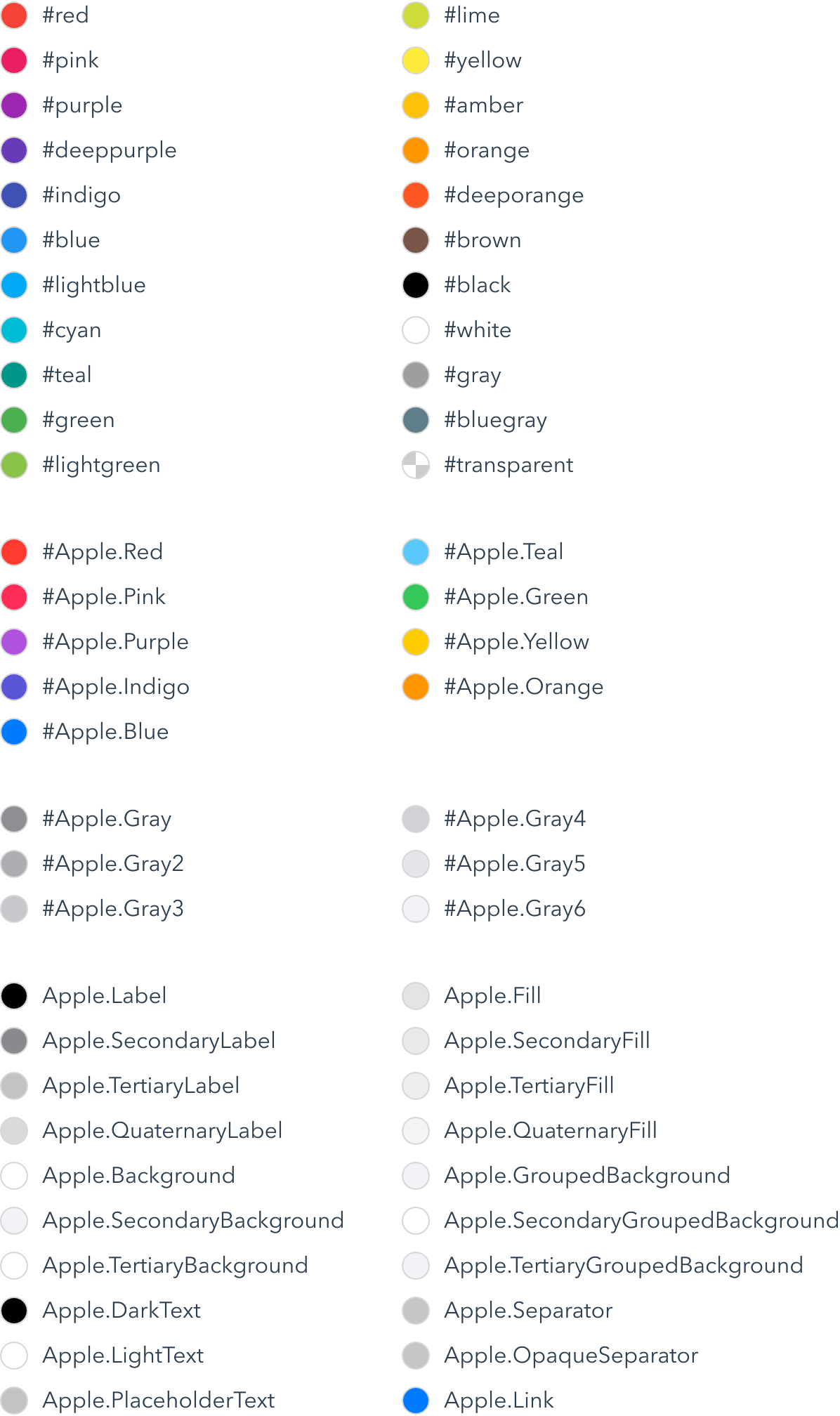
You can define custom color names in your project's "Fonts and Colors" section. You can also specify a color explicitly using either a six or eight character hexadecimal RGB or RGBA format string.
property Color grayColor: #a3a3a3 // RGB property Color transparentBlue: #0000FF7F // RGBA property Color backgroundColor: #blue // Color name
property Float hslHueread only
property Float hslSaturationread only
property Float hslLightnessread only
Returns the hue, saturation and lightness component of the color in HSL color space.
property Float hsvHueread only
property Float hsvSaturationread only
property Float hsvValueread only
Returns the hue, saturation and value component of the color in HSV color space.
static function Color.mix(Color color1, Color color2, Float amount) → Color
static function Color.tween(Color color1, Color color2, Float amount) → Color
Returns a linear blend of color2 into color1. That is, color1 * (1 - amount) + color2 * amount for each of the red, green, blue and alpha channels.
Tween behaves the same as mix, unless either color1 or color2 are #transparent. In this case, tween only interpolates the alpha channel, preserving the red, green and blue channels of the non-transparent color. This is usually the visual appearance you want went tweening between transparent and another color.
static function Color.rgb(Float red, Float green, Float blue) → Color
static function Color.rgb(Float red, Float green, Float blue, Float alpha) → Color
Returns a new color from red, green, blue and alpha (RGB) component values. The component values should be between 0 and 1.
Color.rgb(50/255, 198/255, 64/255) Color.rgb(0.2, 0.45, 0.3) Color.rgb(0.2, 0.45, 0.3, 0.7)
static function Color.hsl(Float hue, Float saturation, Float lightness) → Color
static function Color.hsl(Float hue, Float saturation, Float lightness, Float alpha) → Color
Returns a new color from the HSL hue, saturation and lightness values. The hue value should be between 0 and 360, and the saturation, lightness and alpha values between 0 and 1.
static function Color.hsv(Float hue, Float saturation, Float value) → Color
static function Color.hsv(Float hue, Float saturation, Float value, Float alpha) → Color
Returns a new color from the HSV hue, saturation and value values. The hue value should be between 0 and 360, and the saturation, value and alpha values between 0 and 1.
static function Color.hex(String hex) → Color
Returns a new color from the specified hex color string, in either rgb or rgba form. An optional leading '#' character is allowed.
Color.hex("#f09") // #FF0099 Color.hex("#FFAA99B8")
static function Color.darken(Color color, Float amount) → Color
Returns a copy of color, with the lightness in the HSL color space decreased by the absolute amount. The amount is a value between 0 and 1.
Color.darken(#orange, 0.3) Color.darken(Color.rgb(.3,.4,.7), 0.3)
static function Color.lighten(Color color, Float amount) → Color
Returns a copy of color, with the lightness in the HSL color space increased by the absolute amount. The amount is a value between 0 and 1.
Color.lighten(#orange, 0.3) Color.lighten(Color.rgb(.3,.4,.7), 0.3)
static function Color.saturate(Color color, Float amount) → Color
Returns a new color value. Increase the saturation of a color in the HSL color space by an absolute amount.
Color.saturate(#e3cbb8, 0.3) Color.saturate(Color.rgb(.3,.4,.7), 0.3)
static function Color.desaturate(Color color, Float amount) → Color
Returns a new color value. Decrease the saturation of a color in the HSL color space by an absolute amount.
Color.desaturate(#orange, 0.3) Color.desaturate(Color.rgb(.3,.4,.7), 0.3)