Float
Float is a value type that can store a signed, 64-bit number. That is, a fractional number between approximately -10^308 and 10^308.
If you only need to store whole numbers, consider using Int instead.
Literal syntax
A Float literal can be written directly.
property Float myFloat: 0.2 property Float pi: 3.14159265359 property Float salaryIncrease: -20.3456
When expressing particularly large or small values, it may be more convenient to use exponential notation.
property Float plankTime: 5.391e-44 property Float ageOfUniverse: 4.361e+17
function format(String formatPattern) → String
Returns the float formatted as a string using the format pattern.
The pattern string may contain the following tokens.
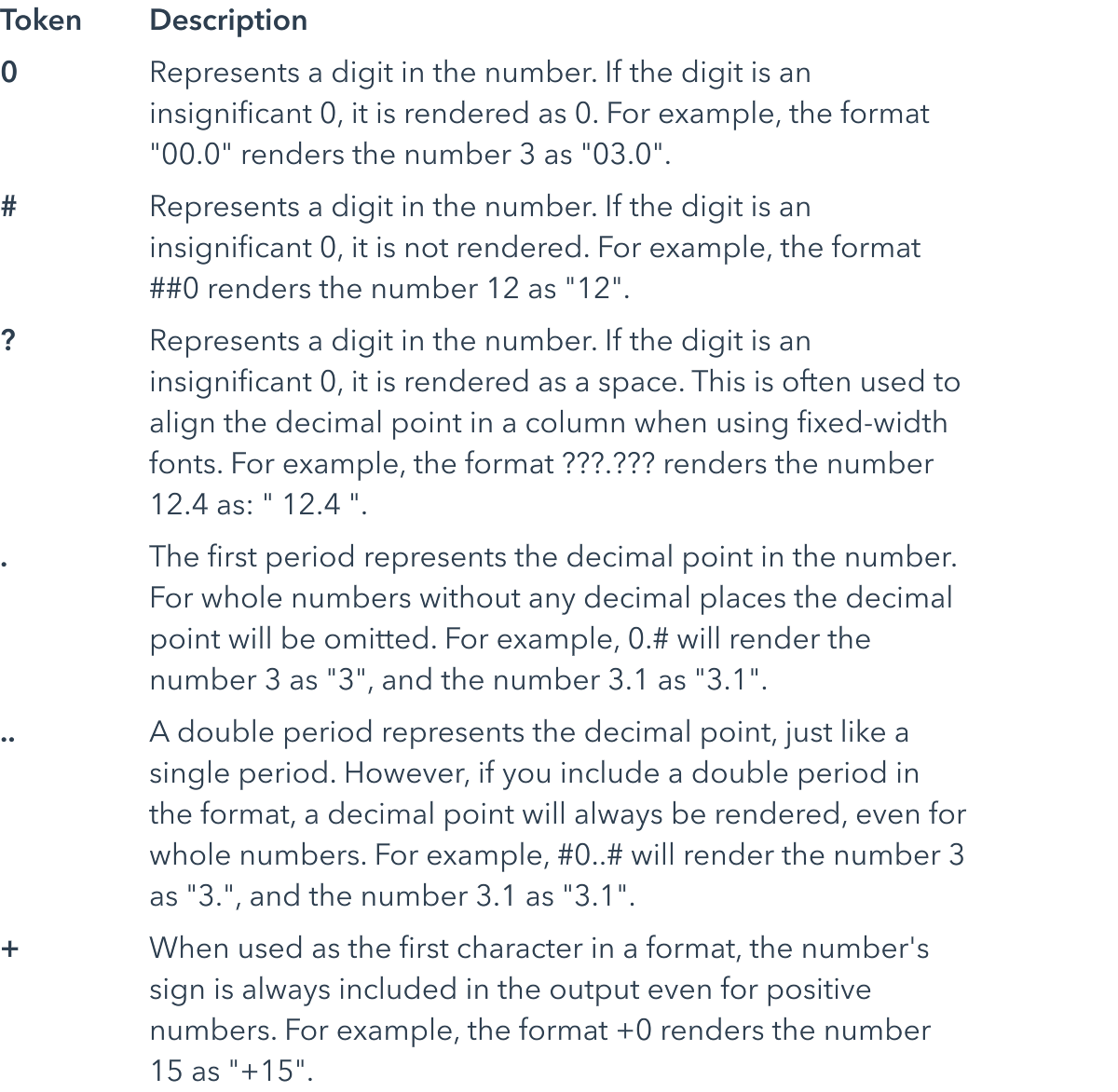
Characters in the pattern that do not form part of a token are left as is. However, as new tokens may be added in the future, it is safest to enclose these "pass through" characters in single quotes to ensure they are never treated as a pattern token.
You can try out formatters and see the results below.

Here are some examples to use as a guide.
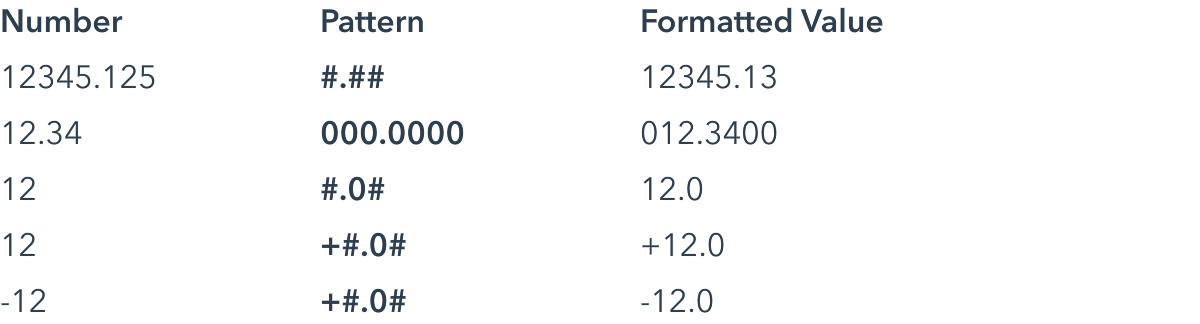